These days I devote my coding time to this small project where I decided to put together the one product e-shop which will be part of my portfolio. I decided to come up with this small project related to my father’s apprenticeship. His project has a name Pecho Crystal and I am building a one-product e-shop for the whisky set product. I wrote down my goals within this project in the first article related to this topic: Coding eCommerce project: what do I want to build? part I. In this article, I would like to cover my starting point within this project. I will link the project GitHub link to the HTML, CSS, and JavaScript code at the end of this article.
What I have already built
Right now I could tell I am almost in the middle of the whole project. I have already built a simple home page and product site.
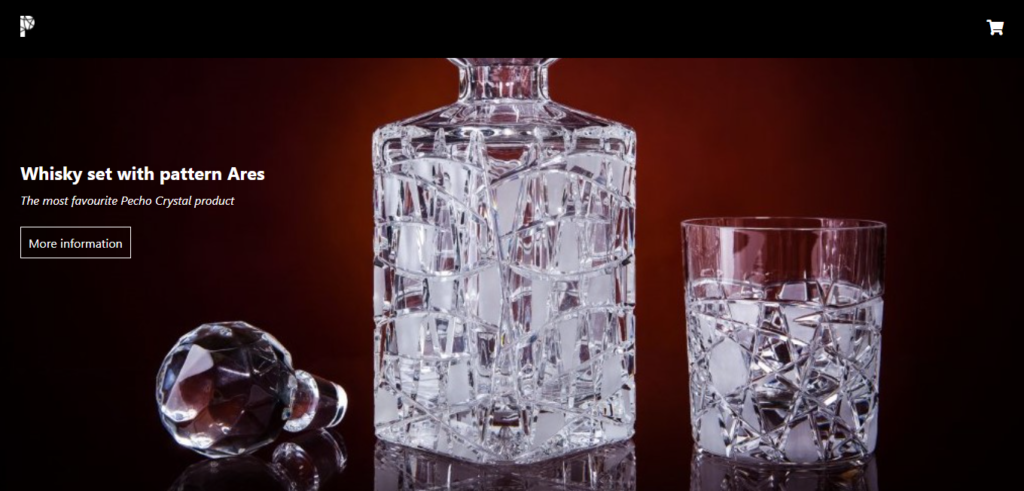
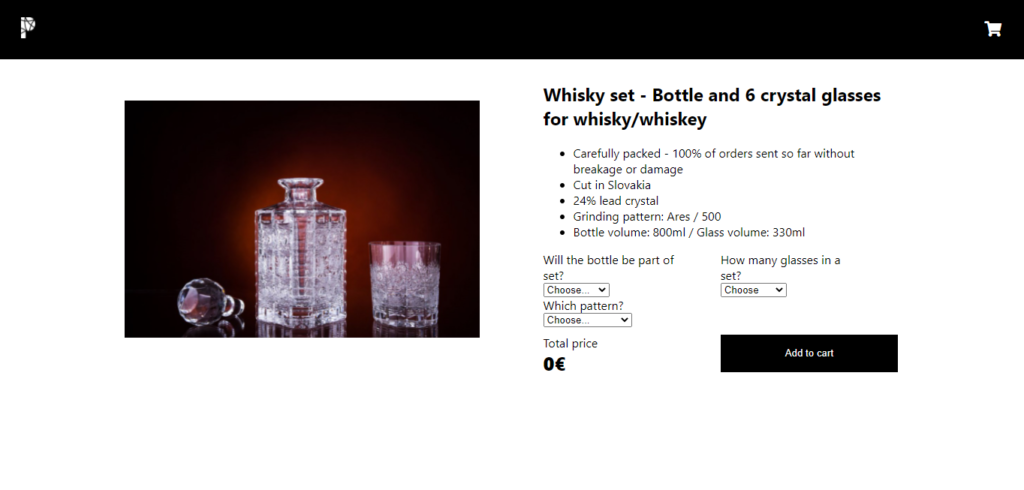
Problems that I solved
One of the problems I needed to solve was that I wanted to be as close as possible to the client. I know from the experience that clients usually like buying whisky sets (that is why I chose this product for this portfolio project), but they also prefer different setups to decide on the whisky sets. Somebody wants just a bottle, somebody just the glasses. If someone decides on the whole set (bottle and glass together) they usually want to choose the number of glasses – 2 or 6 in the set. What we offer extra is some customization on the bottle where we can put initials of the person who will get this special present – usually 2 letters (the first letter of the first name and the first letter of the second name).
Using the <select> in HTML
For making the 4 drop-down menus where the potential customer could choose their preferences I chose to use the HTML tag <select> where I put options with value attributes, which is then used in the JavaScript code. When using the <select> tag it is not possible to use the placeholder attribute, but you can still achieve the effect that there will be a default value hold when the potential customer comes to the website. How to achieve it? As you can see in the snippet below one of the <select> options is for choosing if the whisky bottle will the part of the set. The options are YES or NO, but there is one option that has the role of so-called “placeholder”. To act as a placeholder we create the third option but include also the attributes disabled selected hidden. These 3 words will make the option not visible in the dropdown list and visible as the main option at the beginning before choosing from the drop-down. We can leave the value of this option empty.
<select id="whiskybottle" name="whiskybottle">
<option value="" disabled selected hidden>Choose...</option>
<option value="yes">YES</option>
<option value="no">NO</option>
</select>
What type of event to use for <select>?
Until now I was used to using just one type of event ‘click’. So particular function was executed when I clicked the chosen element (e.g. button). For the <select> HTML tag the ‘click’ event was not the best choice to be made, because the function is executed very early when clicking on the drop-down list. I decided to go with the ‘change’ event.
According to MDN documentation ‘change’ event fires at the moment when the user commits the change explicitly (e.g., by selecting a value from an <select>
‘s dropdown with a mouse click.
Let’s have a look at one of the examples from the code. One of the features I wanted to have within the product site was that after choosing the pattern of the whisky set the product photo will change for the particular photo showing the pattern. So I needed to get elements with the images and I did it by Id and also get the <select> element. In the function changePicture() I used the if-else statement where the condition was based on comparing which pattern was chosen. If the value chosen from the drop-down list is in productPattern “Ares”, a class “displaypicture” will be added to the image that represents the pattern “500”. This will ensure that the picture for pattern “500” will disappear as in the CSS the class “displaypicture” includes a property display and value none. “Displaypicture” class will be also removed from the classList of the image element. If the productPattern. value is not “Ares” but “500” the code will execute the logic vice versa – display the image for “500” and hide the code for “Ares”.
let ares = document.getElementById('ares');
let fiveHundred = document.getElementById('fivehundred');
let productPattern = document.getElementById('productpattern');
function changePicture() {
if (productPattern.value === "Ares") {
fiveHundred.classList.add('displaypicture');
ares.classList.remove('displaypicture');
} else {
ares.classList.add('displaypicture');
fiveHundred.classList.remove('displaypicture');
}
}
productPattern.addEventListener('change', changePicture);
How to show and hide the entire element or section?
As you can see in the picture above as default there are 3 drop-down menus to choose the right combination of productscustomers. There is also the fourth drop-down list but this is hidden by default. Only when I choose that I want a whisky bottle the drop-down will appear and customer can choose if they would like to add some customization on the bottle in the form of initials. (the first letter of the first and the last name)
let customInitials = document.getElementById('custominitials');
let customText = document.getElementById('customtext');
function enableInitialsSection() {
if(whiskyBottle.value ==="yes") {
custom.classList.remove("displaynone");
} else {
custom.classList.add("displaynone");
}
}
function enableInitialsField() {
if(customInitials.value ==="yes") {
customText.classList.remove("displaynone");
} else {
customText.classList.add("displaynone");
}
}
whiskyBottle.addEventListener('change', enableInitialsSection);
customInitials.addEventListener('change', enableInitialsField);
As you can see in the code above when the event ‘change’ occurs on particular elements the functions are executed. With the use of the classList and add/remove method it is possible to add or remove the class ‘displaynone’ from the classList of that particular dropdown. So when the customer wants a bottle he could then also choose if he wants initials on the bottle. If he wants the initials on the bottle that he can enter it into the small box that appears after choosing the option yes I want the initials.
Choosing the options from drop-downs has an impact on the price – how to update it step by step?
The thing is that the potential customer can model his own whisky set and he is not doing it just by choosing the one variant. He is choosing from 4 different drop-downs where 3 of them have an impact on the price. So to show the total price without shipping and payment options I needed to come up with some solution. This is my point of view on it.
let whiskyBottle = document.getElementById('whiskybottle');
let whiskyGlasses = document.getElementById('whiskyglasses');
let customInitials = document.getElementById('custominitials');
let totalPrice = document.getElementById('totalprice');
let totalFinalPrice = document.getElementById('totalfinalprice');
let sumFinalPrice = 0;
let totalProductPrice = 0;
let allThePrices = [];
productPriceList = {
whiskybottleprice0: 0,
whiskybottleprice: 100,
whiskyglassesprice0: 0,
whiskyglassesprice2: 40,
whiskyglassesprice6: 109,
custominitialsprice0: 0,
custominitialsprice: 15,
};
function calculateThePrice() {
sumFinalPrice = allThePrices.reduce((total, item) => {
return total + item
}, 0);
}
function productPartPrice() {
let whiskyBottlePrice;
if(whiskyBottle.value === "yes"){
whiskyBottlePrice = totalProductPrice =+ parseInt(productPriceList.whiskybottleprice);
} else {
whiskyBottlePrice = totalProductPrice = parseInt(productPriceList.whiskybottleprice0);
customInitials.value="";
customText.value="";
};
let whiskyGlassesPrice;
if(whiskyGlasses.value === "2pcs") {
whiskyGlassesPrice = totalProductPrice =+ parseInt(productPriceList.whiskyglassesprice2);
} else if (whiskyGlasses.value === "6pcs"){
whiskyGlassesPrice = totalProductPrice =+ parseInt(productPriceList.whiskyglassesprice6);
} else {
whiskyGlassesPrice = totalProductPrice = parseInt(productPriceList.whiskyglassesprice0);
}
let whiskyBottleInitialsPrice;
if(customInitials.value === "yes") {
whiskyBottleInitialsPrice = totalProductPrice =+ parseInt(productPriceList.custominitialsprice);
} else {
whiskyBottleInitialsPrice = totalProductPrice =+ parseInt(productPriceList.custominitialsprice0);
};
allThePrices[0] = whiskyBottlePrice;
allThePrices[1] = whiskyGlassesPrice;
allThePrices[2] = whiskyBottleInitialsPrice;
calculateThePrice();
totalFinalPrice.innerHTML = sumFinalPrice;
console.log(allThePrices);
console.log(sumFinalPrice);
}
whiskyBottle.addEventListener('change', productPartPrice);
whiskyGlasses.addEventListener('change', productPartPrice);
customInitials.addEventListener('change', productPartPrice);
Firstly I created an object that holds the prices for different variations. For example, if the customer chooses that there will be no bottle within the set the price is 0. (In object under the key-value pair: whiskybottleprice0: 0). There are all of the possible choices from the drop-downs that have some kind of impact on price change.
function productPartPrice() executes the following code. It is triggered by the change in <select> drop-down menu elements which we got and saved in variables whiskyBottle, whiskyGlasses, and customInitials. When there is a change in this element the function starts and the first thing that it will do is to evaluate what value is chosen using the if-else statements. The results of this evaluation are stored in variables let whiskyBottlePrice, whiskyGlassesPrice, whiskyBottleInitialsPrice which are local. After this evaluation, we assign the value to the array with the name allThePrices.
allThePrices[0] = whiskyBottlePrice;
allThePrices[1] = whiskyGlassesPrice;
allThePrices[2] = whiskyBottleInitialsPrice;
The numbers in the brackets are the indexes within the array where we would like to put the values from the variables. After adding these numbers to an array I call another function calculateThePrice() where I use the Array method reduce(), which simply creates at the end “sum” of all elements within the array. Thanks to this we get the whole price which consists of 3 prices (for the bottle, for glasses, and for initials).
At the end we change the price value: totalFinalPrice.innerHTML = sumFinalPrice;
We could console log the array or the sumFinalPrice for checking how it works in the console.
How to add product into the cart?
In this phase, we already have the necessary functionality to get the whole price for the product. What we need to think about is also the way how we approach saving all the info from <select> tags in a way that we could use it later in e.g. shopping cart etc.
As this e-shop is just for one product it does not mean that the user cannot choose more than one configuration of sets. That is why I decided to define class Product which is the fingerprint for all product configurations. This is triggered every time the potential customer hits the Add to cart button.
As you see in the code below, after clicking on Add to cart button there will be an evaluation of whether the customer has chosen all the necessary variants. There is an alert message if he does not fill either of the variants or choose just yes for the whisky bottle without the initials. Then the alert is applicable the function ends and no product is submitted Customer needs to close the alert window and choose all the necessary variants.
If everything is correctly chosen new product will be created and stored in variable newProductItem which will be then pushed to the Array called productList. There is one more evaluation and I take the pattern that they choose and differ between these 2 values because there is a different output in the case of the picture. So based on their pattern choice, a different picture is chosen. For our control, we can console.log(productList) to see if all the required values are available. We need to also clear the allThePrices array. After click on Add To Cart button we also need to call the function enableAddToCartOverlay().
class Product {
constructor(picture, whiskyBottle, whiskyGlasses, productPattern, customInitials, customText, price) {
this.picture = picture;
this.whiskyBottle = whiskyBottle;
this.whiskyGlasses = whiskyGlasses;
this.productPattern = productPattern;
this.customInitials = customInitials;
this.customText = customText;
this.price = price;
}
};
function newProduct() {
if ((whiskyBottle.value === "" || whiskyGlasses.value === "" || productPattern.value === "") || (whiskyBottle.value === "yes" && customInitials.value === "")) {
alert('Please choose the options of your set!');
return;
} else if (productPattern.value === 'Ares') {
const newProductItem = new Product(ares.src, whiskyBottle.value, whiskyGlasses.value, productPattern.value, customInitials.value, customText.value, sumFinalPrice);
productList.push(newProductItem);
} else {
const newProductItem = new Product(fiveHundred.src, whiskyBottle.value, whiskyGlasses.value, productPattern.value, customInitials.value, customText.value, sumFinalPrice);
productList.push(newProductItem);
}
console.log(productList);
allThePrices = [];
enableAddToCartOverlay();
}
function enableAddToCartOverlay() {
addToCartOverlay.classList.remove('displaynone');
}
function disableAddToCartOverlay() {
addToCartOverlay.classList.add('displaynone');
allThePrices = [];
whiskyBottle.value="";
whiskyGlasses.value="";
productPattern.value="";
customInitials.value="";
customText.value="";
totalFinalPrice.innerHTML=0;
enableInitialsSection();
}
addToCartButton.addEventListener('click', newProduct);
continueShopping.addEventListener('click', disableAddToCartOverlay);
addToCartOverlay.addEventListener('click', disableAddToCartOverlay);
enableAddToCartOverlay() removes the ‘displaynone’ class from the addToCartOverlay class list and screen overlay with the message that the product was added to the cart and 2 buttons are shown. The first button says – Continue shopping and the second button says go to the cart. Another function disableAddToCartOverlay() is called after clicking on Continue shopping or the grey, transparent background. This function adds the class ‘displaynone’ back, clears the allThePrices array, and also clears all the drop-down <select> elements to their default values. The total price is also put back to zero.
Questions I have
As I am in the learning phase of OOP I am trying my best to understand it and implement it in the best way possible. I already come up with some specific solutions for Shopping carts and Orders. I will see if my presumptions would work also in a practical way. The main question for me still is as I am building a multiple-page one-product e-shop website how can I keep the data in the cart within the whole ordering process. I assume that I would either use local storage or probably create some cookies? I need to dive into this problem.
How will I continue?
I plan to build the Shopping Cart and the whole order process with shipping, payment options, and also invoice addresses. The last step will be to implement the feature that will send out the email with all the details to the customer’s email address and e-shop email address. So these are the next steps that I would like to bring to this small portfolio project.
References for you to discover more
<select> reduce() array if-else statement class constructor function event: change
You can find the whole code HTML, CSS and JavaScript on this GitHub repository.
Follow me on socials or write me an email or message on Linkedin
Sorry for any mistakes. If you find any please let me know. You can find my contact info down below.
I am just a beginner. Do not take this as final and the only solution. I am just sharing my learning journey. If there are any advices related to code shoot me an email.
Photo used in this article is from Pecho Crystal
Photo on cover by Carlos Muza on Unsplash